Nếu bạn đang tìm cách xây dựng một ứng dụng với Vue 3 JS và Laravel và bạn muốn triển khai chức năng CURD thì sau đây tôi sẽ hương dẫn các bạn làm việc đó.
Trong hướng dẫn này bạn sẽ học cách thiết lập Laravel 10 và Vue.js để triển khai chức năng CURD.
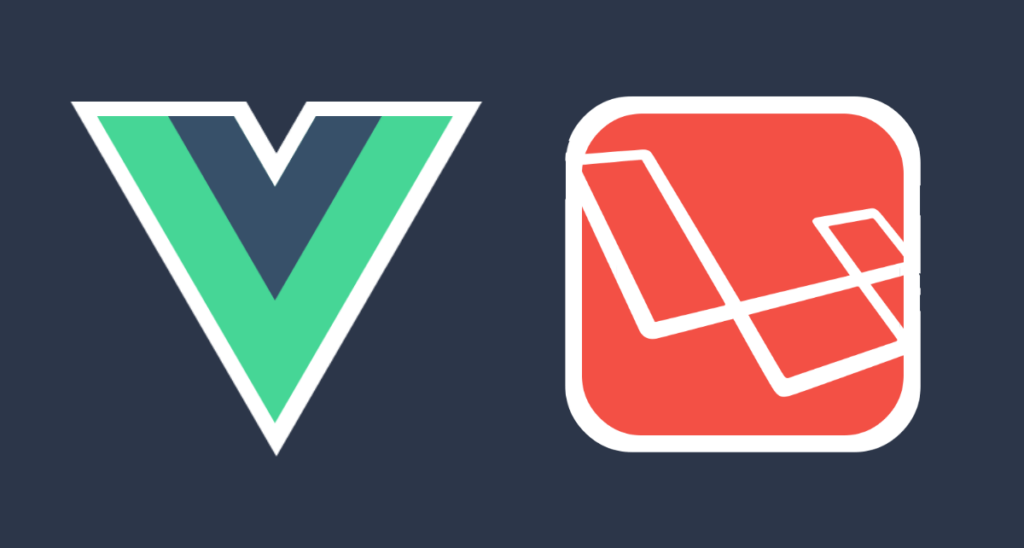
Cách tạo ứng dụng CRUD trong Laravel 10 Vue 3 JS
- Bước 1: Thiết lập ứng dụng Laravel 10
- Bước 2: Cấu hình cơ sở dữ liệu
- Bước 3: Cài đặt và cấu hình Vue.js
- Bước 4: Tạo Migration, Model và Controller
- Bước 5: Xác định router API
- Bước 6: Tạo ứng dụng Vue Js
- Bước 7: Tạo các thành phần Vue.js
- Bước 8: Xác định router Vue Js cho ứng dụng Crud
- Bước 9: Đưa Vue Js Dependencies vào app.js
- Bước 10: Chạy ứng dụng
Bước 1: Thiết lập ứng dụng Laravel 10
Trước hết, hãy mở comamnd của bạn.
Sau đó thực hiện lệnh sau để tải xuống thiết lập mới laravel:
composer create-project --prefer-dist laravel/laravel blog
Bước 2: Cấu hình cơ sở dữ liệu
Khi bạn đã cài đặt laravel 10. Sau đó, bạn cần cấu hình cơ sở dữ liệu
Vì vậy, hãy truy cập vào thư mục gốc ứng dụng Laravel 10 đã tải xuống và mở tệp .env. Sau đó thêm chi tiết cơ sở dữ liệu như sau:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=here your database name here
DB_USERNAME=here database username here
DB_PASSWORD=here database password here
Bước 3: Cài đặt và cấu hình Vue.js
Trong bước này, thực hiện lệnh sau trên comamnd để cài đặt frontend dependencies:
npm install
Tiếp theo, cài đặt vue-router và vue-axios. vue-axios sẽ được sử dụng để gọi Laravel API. Thực hiện lệnh sau:
npm install vue-router vue-axios --save
Sau khi cài đặt tất cả các gói thư viện, bạn chạy lệnh sau:
npm run watch
Lệnh này sẽ lắng nghe các thay đổi tệp và sẽ biên dịch ngay lập tức. Bạn cũng có thể thực hiện lệnh npm run dev nhưng nó sẽ không lắng nghe những thay đổi của bạn
Bước 4: Tạo Migration, Model và Controller
Tạo model Post, migration và controller. Vì vậy, hãy thực hiện lệnh sau:
php artisan make:model Post -m
Bây giờ hãy mở tệp create_posts_table.php từ database>migrations và thay thế hàm up() bằng hàm này:create_posts_table.php
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('title');
$table->text('description');
$table->timestamps();
});
}
Tiếp theo chúng ta sẽ thực hiện migrations bằng lệnh sau
php artisan migrate
Tiếp theo, mở file model Post.php , được đặt trong thư mục app/models và cập nhật code vào Post.php model:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
use HasFactory;
protected $fillable = [
'title', 'description'
];
}
Bây giờ, mở Command của bạn và thực hiện lệnh sau để tạo tập tin PostController.php:
php artisan make:controller API\PostController
Tiếp theo mở PostController và định nghĩa index, add, edit, delete method trong file PostController. Vì vậy, vào thư mục app>Http>Controllers>API và mở tập tin PostController.php. Sau đó cập nhật nó như sau:
<?php
namespace App\Http\Controllers\API;
use App\Http\Controllers\Controller;
use App\Models\Post;
use Illuminate\Http\Request;
use Validator;
class PostController extends Controller
{
// all posts
public function index()
{
$posts = Post::all()->toArray();
return array_reverse($posts);
}
// add post
public function add(Request $request)
{
$post = new Post([
'title' => $request->input('title'),
'description' => $request->input('description')
]);
$post->save();
return response()->json('The post successfully added');
}
// edit post
public function edit($id)
{
$post = Post::find($id);
return response()->json($post);
}
// update post
public function update($id, Request $request)
{
$post = Post::find($id);
$post->update($request->all());
return response()->json('The post successfully updated');
}
// delete post
public function delete($id)
{
$post = Post::find($id);
$post->delete();
return response()->json('The post successfully deleted');
}
}
Bước 5: Xác định router API
Bây giờ chúng ta định nghĩa router web.php và api.php. Vì vậy, hãy vào thư mục Routes và Mở tệp web.php và Api.php và cập nhật các router sau:
Routes/web.php
<?php
Route::get('{any}', function () {
return view('layouts.app');
})->where('any', '.*');
Bây giờ hãy mở tệp api.php và thêm các router vào:
Route::get('posts', 'PostController@index');
Route::group(['prefix' => 'post'], function () {
Route::post('add', 'PostController@add');
Route::get('edit/{id}', 'PostController@edit');
Route::post('update/{id}', 'PostController@update');
Route::delete('delete/{id}', 'PostController@delete');
});
Bước 6: Tạo ứng dụng Vue Js
Trong bước này, di chuyển đến resources/views và tạo một thư mục có tên layouts. Bên trong thư mục này, tạo một tệp có tên là tệp app.blade.php
<!doctype html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" value="{{ csrf_token() }}"/>
<title>Laravel 10 Vue JS CRUD Example - Tutsmake</title>
<link href="https://fonts.googleapis.com/css?family=Nunito:200,600" rel="stylesheet" type="text/css">
<link href="{{ mix('css/app.css') }}" type="text/css" rel="stylesheet"/>
<style>
.bg-light {
background-color: #eae9e9 !important;
}
</style>
</head>
<body>
<div id="app">
</div>
<script src="{{ mix('js/app.js') }}" type="text/javascript"></script>
</body>
</html>
Bước 7: Tạo các thành phần Vue.js
Trong bước này, vào thư mục resources->js và tạo các tệp thành phần vue js sau:
- App.vue
- AllPosts.vue
- AddPost.vue
- EditPost.vue
Lưu ý rằng, App.vue là tệp Vue chính. Chúng ta sẽ định nghĩa router- view trong tệp. Vì vậy, tất cả các điều hướng trang sẽ được hiển thị trong tệp App.vue
Mở tệp App.vue và cập nhật mã sau vào tệp của bạn:
<template>
<div class="container">
<div class="text-center" style="margin: 20px 0px 20px 0px;">
<span class="text-secondary">Laravel Vue CRUD Example</span>
</div>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<div class="collapse navbar-collapse">
<div class="navbar-nav">
<router-link to="/" class="nav-item nav-link">Home</router-link>
<router-link to="/add" class="nav-item nav-link">Add Post</router-link>
</div>
</div>
</nav>
<br/>
<router-view></router-view>
</div>
</template>
<script>
export default {}
</script>
Tiếp theo, Mở tệp AllPosts.vue và cập nhật mã sau vào tệp của bạn:
<template>
<div>
<h3 class="text-center">All Posts</h3><br/>
<table class="table table-bordered">
<thead>
<tr>
<th>ID</th>
<th>Title</th>
<th>Description</th>
<th>Created At</th>
<th>Updated At</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="post in posts" :key="post.id">
<td>{{ post.id }}</td>
<td>{{ post.title }}</td>
<td>{{ post.description }}</td>
<td>{{ post.created_at }}</td>
<td>{{ post.updated_at }}</td>
<td>
<div class="btn-group" role="group">
<router-link :to="{name: 'edit', params: { id: post.id }}" class="btn btn-primary">Edit
</router-link>
<button class="btn btn-danger" @click="deletePost(post.id)">Delete</button>
</div>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
posts: []
}
},
created() {
this.axios
.get('http://localhost:8000/api/posts')
.then(response => {
this.posts = response.data;
});
},
methods: {
deletePost(id) {
this.axios
.delete(`http://localhost:8000/api/post/delete/${id}`)
.then(response => {
let i = this.posts.map(item => item.id).indexOf(id); // find index of your object
this.posts.splice(i, 1)
});
}
}
}
</script>
Tiếp theo, Mở tệp AddPost.vue và cập nhật mã sau vào tệp của bạn:
<template>
<div>
<h3 class="text-center">Add Post</h3>
<div class="row">
<div class="col-md-6">
<form @submit.prevent="addPost">
<div class="form-group">
<label>Title</label>
<input type="text" class="form-control" v-model="post.title">
</div>
<div class="form-group">
<label>Description</label>
<input type="text" class="form-control" v-model="post.description">
</div>
<button type="submit" class="btn btn-primary">Add Post</button>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
post: {}
}
},
methods: {
addPost() {
this.axios
.post('http://localhost:8000/api/post/add', this.post)
.then(response => (
this.$router.push({name: 'home'})
// console.log(response.data)
))
.catch(error => console.log(error))
.finally(() => this.loading = false)
}
}
}
</script>
Tiếp theo, mở tệp EditPost.vue và cập nhật mã sau vào tệp của bạn:
<template>
<div>
<h3 class="text-center">Edit Post</h3>
<div class="row">
<div class="col-md-6">
<form @submit.prevent="updatePost">
<div class="form-group">
<label>Title</label>
<input type="text" class="form-control" v-model="post.title">
</div>
<div class="form-group">
<label>Description</label>
<input type="text" class="form-control" v-model="post.description">
</div>
<button type="submit" class="btn btn-primary">Update Post</button>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
post: {}
}
},
created() {
this.axios
.get(`http://localhost:8000/api/post/edit/${this.$route.params.id}`)
.then((response) => {
this.post = response.data;
// console.log(response.data);
});
},
methods: {
updatePost() {
this.axios
.post(`http://localhost:8000/api/post/update/${this.$route.params.id}`, this.post)
.then((response) => {
this.$router.push({name: 'home'});
});
}
}
}
</script>
Bước 8: Xác định router Vue Js cho ứng dụng Crud
Bây giờ, bạn cần xác định router Vue. Vì vậy, hãy vào thư mục resources>js, tạo một tệp có tên routes.js và cập nhật các route sau vào routes.js file của bạn:
import AllPosts from './components/AllPosts.vue';
import AddPost from './components/AddPost.vue';
import EditPost from './components/EditPost.vue';
export const routes = [
{
name: 'home',
path: '/',
component: AllPosts
},
{
name: 'add',
path: '/add',
component: AddPost
},
{
name: 'edit',
path: '/edit/:id',
component: EditPost
}
];
Bước 9: Đưa Vue Js Dependencies vào app.js
Bây giờ, bạn cần thêm tất cả các routes, vue axios và các phụ thuộc(dependencies) khác, v.v. Hãy chuyển đến thư mục resources>js và mở app.js. Sau đó, cập nhật mã sau vào tệp app.js của bạn:
require('./bootstrap');
import Vue from ‘vue/dist/vue’;
window.Vue = require('vue');
import App from './App.vue';
import VueRouter from 'vue-router';
import VueAxios from 'vue-axios';
import axios from 'axios';
import {routes} from './routes';
Vue.use(VueRouter);
Vue.use(VueAxios, axios);
const router = new VueRouter({
mode: 'history',
routes: routes
});
const app = new Vue({
el: '#app',
router: router,
render: h => h(App),
});
Bước 10: Chạy ứng dụng
npm run watch
Kết thúc
Qua ví dụ trên bạn có thể hiểu được cách hoạt động của Vue.js và Laravel. Hi vọng bạn có thể tạo được ứng dụng CURD của bạn thành công!
Tham khảo các tài liệu về Laravel và Vuejs